Quite often I find myself over at http://thesession.org checking out old traditional tunes. The site offers the tunes as sheet music and ABC Notation. While I certainly appreciate having ready access to the tunes, I find that, more often than not, I need to copy the ABC notation into a text file, convert the text file into a [lilypond[(http://lilypond.org) file, transpose the lilypond file to the G Major scale, and then finally add some code that will display the tune as banjo tabs. Oh man, did you catch all of that? There has to be an easier way to do this.....
Oh, there is...
For the most part, the process is just a series of text manipulations which can be accomplished in just about any programming language. Since I am currently on a Ruby kick, I figured I would just hammer out a quick script to automate the process of retrieving ABC notation from a given page and converting the results into something more usable.
Enter the Ruby
require 'optparse'
require 'open-uri'
require 'rexml/document'
require 'iconv'
def sanitize( string )
#replace non alpha-numerics with _
string = string.gsub(/[^A-z0-9_]/,'_')
return string.downcase
end
def error( string )
puts "**** ERROR ****"
puts string
exit
end
option = {}
OptionParser.new do |opts|
opts.banner = "Usage: sessionconvert [options] [thesession.org_URL]"
opts.on("-b", "--banjo", "add banjo tabulature") do |v|
option[:banjo] = v
end
opts.on("-l", "--lilypond", "compile with lilypond") do |v|
option[:lilypond] = v
end
end.parse!
url = ARGV[0]
# was a url supplied?
unless url
error "you must supply a thesession.org url"
end
begin
text = open(url).read
rescue
error "Failed to read #{url}"
end
begin
#watch out for sneaky
ic = Iconv.new('UTF-8//IGNORE', 'UTF-8')
valid_string = ic.iconv(text)
doc = REXML::Document.new valid_string
rescue
error "Failed to parse #{url}"
end
root = doc.root
p = root.elements["body/div[@id='abc']/div[@class='box']/p"].to_s
abc = p.gsub(/<.*>/,'')
#get the title
m = /T: (?<title>.*)/.match( abc )
title = m['title']
#get the key
m = /K: (?<key>.*)/.match( abc )
key = m['key']
#sanitize the title, we will use this a bunch
sanitized_title = sanitize(title)
#what will the filenames be?
filename_abc = sanitized_title+".abc"
f = open(filename_abc,'w')
f.write(abc)
f.close
begin
`abc2ly #{filename_abc}`
rescue
error "abc2ly is not installed"
end
#what will the lilypond file be?
filename_ly = sanitized_title+".ly"
if option[:banjo]
#what is the key we are transposing from
t_key = key[0].downcase
banjer_bit = "\\transpose #{t_key} g,
\\context TabStaff = \"banjer\"{
\\set TabStaff.stringTunings = #banjo-open-g-tuning
\\set Staff.instrumentName = #\"Banjo\"
\\context TabVoice = \"banjer\" { \\tabFullNotation \\stemDown \\voicedefault }
}"
text = File::read(filename_ly)
#substitute the banjer stuff
text = text.gsub(/<<.*>>/m, "<<\n #{banjer_bit}\n\t>>")
#make a new lilypond file
filename_ly = sanitized_title+"-banjo.ly"
#write the new text to file
f = open(filename_ly,'w')
f.write(text)
f.close
end
#did the user request compiling
begin
`lilypond #{filename_ly}` if option[:lilypond]
rescue
error "lilypond is not installed"
end
If you have problems copying the code, please see http://hoof.jezra.net/snip/nQ
Usage
Since I don't always want to convert a tune to banjo tabs (some tunes are better on the concertina), a flag (-b) needs to be included with the arguments to specify that the tune should be converted to banjo tabs. Similarly, including the -l flag with compile the final lilypond file into a pdf of sheet music or tabs.
For example: The tune Rakes of Mallow is found at http://www.thesession.org/tunes/display/85 and to convert the tune to banjo tabs and compile to a PDF the following command would be used.
This is what the final PDF looks like. Damn that's nice!

...and this is me butchering the tune on my banjo. Damn, I need more practice.
Awesome! Now quit reading, and go butcher a tune on the instrument of your choice.
Due to an unfortunate series of events, Shemp ( my homemade banjo ) is out of commission. However, I recently purchased a used Sovereign banjo that needed a bit of work to get into a somewhat decent playing condition. One thing I really wanted for this banjo was a strap. You know, just in case I want to wander around the block while picking a tune.
For my banjo strap, I wanted something fairly easy to remove and very easy on the wallet. For two bucks spent at the thrift store, and bits of stuff lying around my home, I had a pretty sweet banjer strap in no time.
Four Bottle caps
Beer bottle caps, hot glue, and magnets make great bottle cap fridge magnets, but that's not what I'm making right now. From the bottle cap collection, I selected two Red Tails, a PBR, and a Highlife.
Gettin Hammered!
All four of the caps was hammered flat. The Red Tail caps flattened nice and clean, the other two.... well not so much. Notice that the PBR cap is a Jack of Diamonds? That was intentional.
Stuck Together
The flat caps where paired up and a hole what punched through their centers. To keep things neat and to cover any sharp edges, an eyelet was popped into the center of the stacked caps.
The actual "strap"
Two dollars at Sack's Thrift Avenue purchased a leather belt. Damn, I'm busting the bank on this one. A slit the length of the flat caps diameter was cut into each end of the belt and a small hole was punched for good measure.
Hold on
Some red leather cord was knotted at one end, fed through the cap eyelet, looped through the rim bolt and over the caps to secure the whole thing nicely. It's quite easy to remove and adjust. This was done twice.
Done
"Button" the caps through both ends of the belt, throw that baby over the shoulder and frail some Cripple Creek on the front porch!
It is really weird to play standing up, as I am so accustomed to easily seeing the strings and frets when sitting.
Now quit reading, and go parse the following with lilypond:
\version "2.12.2" \header { title = "~$ sudo modprobe" composer = "Fabian Scherschel & Dan Lynch" copyright = "Creative Commons Attribution-Share-Alike 3.0" } melody = \relative c' { \key e \minor \time 4/4 \numericTimeSignature e4 d8 e4 d8 e[ fis] | % 1 g2. b4 | % 2 a2. r8 b8 ~ | % 3 b8 a g a g a g fis | % 4 e4 d8 e4 d8 e[ fis] | % 5 g2. ~ g8 fis | % 6 d1 ~ | % 7 d2 r | % 8 \bar "|." } \score { \transpose c' c \context TabStaff = "banjer" << \set TabStaff.stringTunings = #banjo-open-g-tuning \set Staff.instrumentName = #"Banjo" \context TabVoice = "banjer" { \melody } >> \layout {} }
...and thank you Dr. Kulp
ha, nice idea for a strap, jez. how come i get the feeling, if left to your own devices, in a few years you will be living in a house made of choice recyclables and duct tape?
;)
Sorry it took so long to respond...had a hard time figuring out the math problem to prove my humanness.
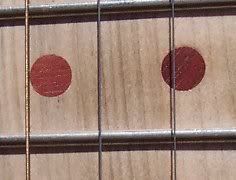
A forchner bit drilled the hole in the neck and a plug bit cut plugs of blood wood that I gently tapped into place. Well, it might not have been that gentle and two of the pegs broke just below the level of the neck. Hey! it adds personality.
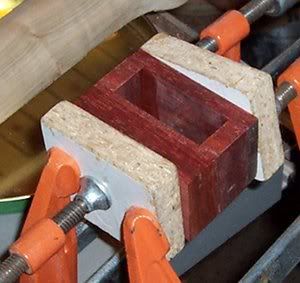
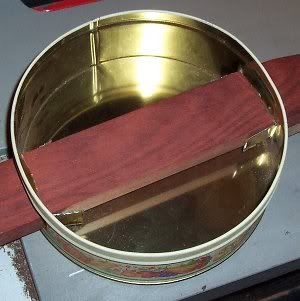
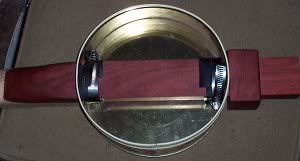
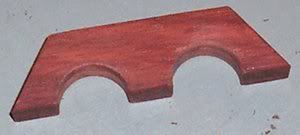
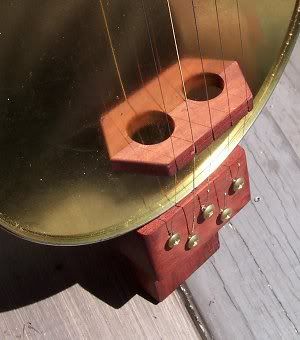
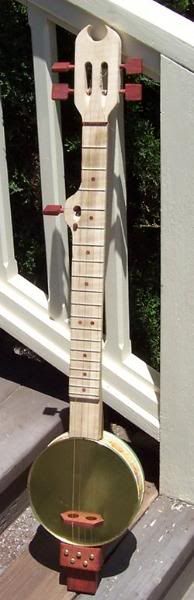
For reason's that most people probably wouldn't understand, she has been affectionately named Shemp in honor of Shemp Howard: my favorite of the six Three Stooges.
After sitting down and taking the time to properly tune Shemp, she sounds quite nice and hopefully, in a few weeks, I'll have gained enough skill on the banjer to make a recording to share (it will be 'Banks and Braes'; I love that tune ).
For the next banjo, should I decide to make another, I'll use a dark wood for the fretboard as it is currently hard to see the strings against the light wood; especially with the grain going with the strings. I would also like to make a wooden pot, although I don't think I'll make a proper banjo head. Hmmm, my buddy does have a lathe.......
I'd like to thank my buddy for the use of his shop, Pat and Patrick Costello for inspiring and teaching me through their blog posts, books, and videos. Frail on!
Well that's all for now, time to get back to banjo practice!
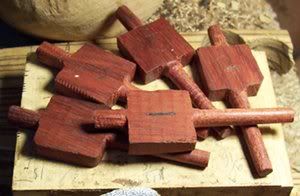
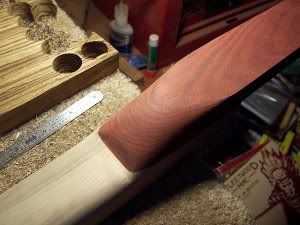
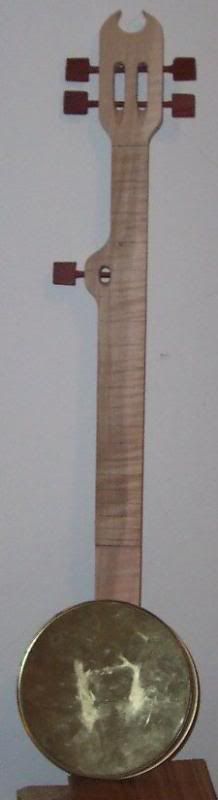
Next up (in theory): fret dot inlays, cutting the pot to fit the potrod, crafting the nut and bridge, figuring out the tailpiece/saddle.
There is a certain level of "how the crap am I going to do that?" when I get to each step of making pieces of the banjer. Sometimes, the problem is solved with a bit of woodshop instruction from my buddy and the use of a jig that he has made. Other times, well, there is probably a tool to do what I need, but it isn't in the woodshop.
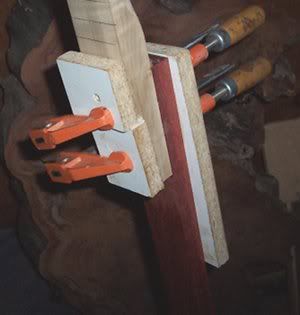
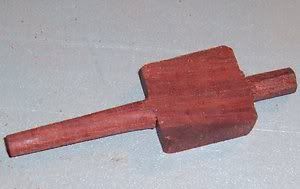
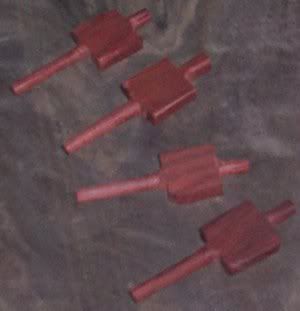
Next up: making the 5th tuning peg, tapering the peg holes on the neck, shaping the neck and potrod, making a nut and bridge and string saddle, attaching the pot to the potrod, learning to play the banjo.
I am a fortunate person with good crafty friends.
A very good friend of mine happens to enjoy crafting wood and he agreed to let me make a banjo in his wood shop during the weekends. First thing first, select a piece of wood for the neck. To be honest, the first thing that I did was to purchase a candy tin from a local thrift store to be the pot of my banjo. Back to the neck. While searching through the scrap wood in the shop I found a piece of maple that my friend said was a poor choice because it was sap wood. Oh the poor rejected piece of wood. Just kidding, I chose the sap wood piece. It was about 35"x3"x1". There were probably a few 16ths, 32nds,8ths, or quarters that should be included in the measurement but I don't like fractions. Metric System; for the win!
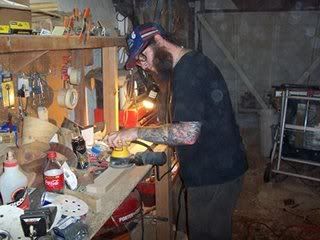
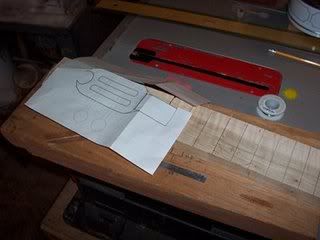
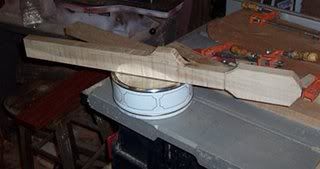
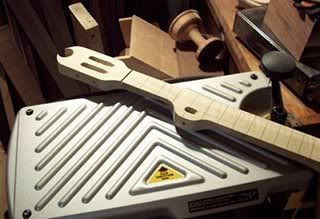
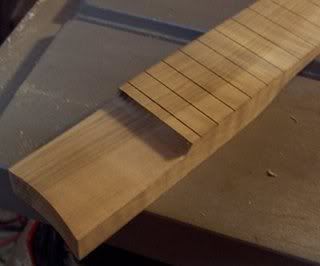
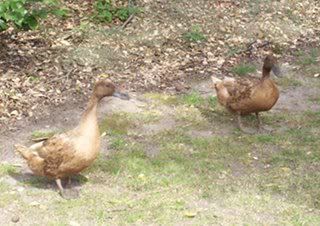
That's it for part 1. Next weekend is tuning pegs and other assorted nick-nacks.